文本到图像
[[open-in-colab]]
当你想到扩散模型时,文本到图像通常是首先想到的事情之一。文本到图像根据文本描述(例如,"丛林中的宇航员,冷色调,柔和的色彩,细节丰富,8k")生成图像,这也称为提示。
从非常高的层面上讲,扩散模型接收提示和一些随机初始噪声,并迭代地去除噪声以构建图像。去噪过程由提示引导,一旦去噪过程在预定的时间步数后结束,图像表示就会被解码成图像。
你可以在 🤗 Diffusers 中通过两个步骤从提示生成图像:
- 将检查点加载到 [
AutoPipelineForText2Image
] 类中,该类会根据检查点自动检测要使用的适当管道类:
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16, variant="fp16"
).to("cuda")
请提供英文内容,我会尽力将其翻译成符合要求的中文。
image = pipeline(
"stained glass of darth vader, backlight, centered composition, masterpiece, photorealistic, 8k"
).images[0]
image
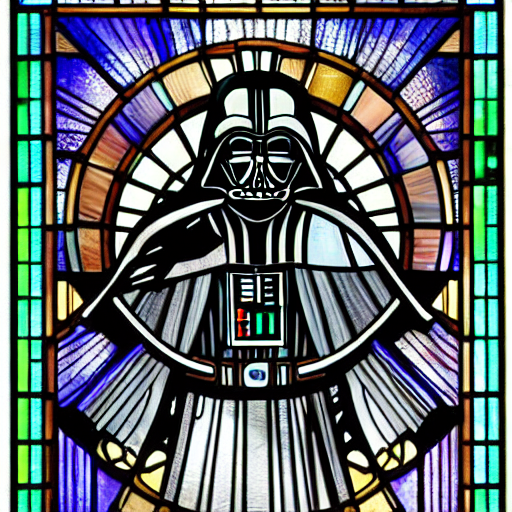
热门模型
最常见的文本到图像模型包括 Stable Diffusion v1.5、Stable Diffusion XL (SDXL) 和 Kandinsky 2.2。此外,还有 ControlNet 模型或适配器,可以与文本到图像模型一起使用,以便在生成图像时进行更直接的控制。由于模型的架构和训练过程不同,每个模型的结果略有不同,但无论你选择哪个模型,它们的用法都大致相同。让我们对每个模型使用相同的提示,并比较它们的结果。
Stable Diffusion v1.5
Stable Diffusion v1.5 是一个潜在扩散模型,它从 Stable Diffusion v1-4 初始化,并在 LAION-Aesthetics V2 数据集的 512x512 图像上进行了 595K 步的微调。你可以像这样使用此模型:
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16, variant="fp16"
).to("cuda")
generator = torch.Generator("cuda").manual_seed(31)
image = pipeline("Astronaut in a jungle, cold color palette, muted colors, detailed, 8k", generator=generator).images[0]
image
Stable Diffusion XL
SDXL 是之前 Stable Diffusion 模型的更大版本,它包含一个两阶段模型过程,可以为图像添加更多细节。它还包括一些额外的微调,以生成以中心主题为中心的优质图像。查看更全面的SDXL指南,了解有关如何使用它的更多信息。一般来说,你可以像这样使用 SDXL:
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stabilityai/stable-diffusion-xl-base-1.0", torch_dtype=torch.float16, variant="fp16"
).to("cuda")
generator = torch.Generator("cuda").manual_seed(31)
image = pipeline("Astronaut in a jungle, cold color palette, muted colors, detailed, 8k", generator=generator).images[0]
image
Kandinsky 2.2
Kandinsky 模型与 Stable Diffusion 模型略有不同,因为它还使用图像先验模型来创建嵌入,这些嵌入用于在扩散模型中更好地对齐文本和图像。
使用 Kandinsky 2.2 最简单的方法是:
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"kandinsky-community/kandinsky-2-2-decoder", torch_dtype=torch.float16
).to("cuda")
generator = torch.Generator("cuda").manual_seed(31)
image = pipeline("Astronaut in a jungle, cold color palette, muted colors, detailed, 8k", generator=generator).images[0]
image
ControlNet
ControlNet 模型是附加模型或适配器,在文本到图像模型(例如 Stable Diffusion v1.5)之上进行微调。将 ControlNet 模型与文本到图像模型结合使用,为更明确地控制图像生成方式提供了多种选择。使用 ControlNet,你可以在模型中添加一个额外的条件输入图像。例如,如果你提供一个人体姿势的图像(通常表示为连接成骨架的多个关键点)作为条件输入,模型将生成一个遵循图像姿势的图像。查看更深入的 ControlNet 指南,以了解有关其他条件输入及其使用方法的更多信息。
在本例中,让我们使用人体姿势估计图像对 ControlNet 进行条件化。加载在人体姿势估计上预训练的 ControlNet 模型:
from diffusers import ControlNetModel, AutoPipelineForText2Image
from diffusers.utils import load_image
import torch
controlnet = ControlNetModel.from_pretrained(
"lllyasviel/control_v11p_sd15_openpose", torch_dtype=torch.float16, variant="fp16"
).to("cuda")
pose_image = load_image("https://huggingface.co/lllyasviel/control_v11p_sd15_openpose/resolve/main/images/control.png")
将 controlnet
传递给 [AutoPipelineForText2Image
],并提供提示和姿势估计图像:
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", controlnet=controlnet, torch_dtype=torch.float16, variant="fp16"
).to("cuda")
generator = torch.Generator("cuda").manual_seed(31)
image = pipeline("Astronaut in a jungle, cold color palette, muted colors, detailed, 8k", image=pose_image, generator=generator).images[0]
image
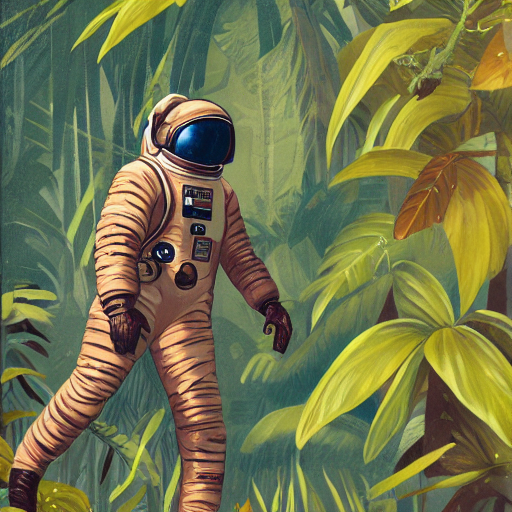
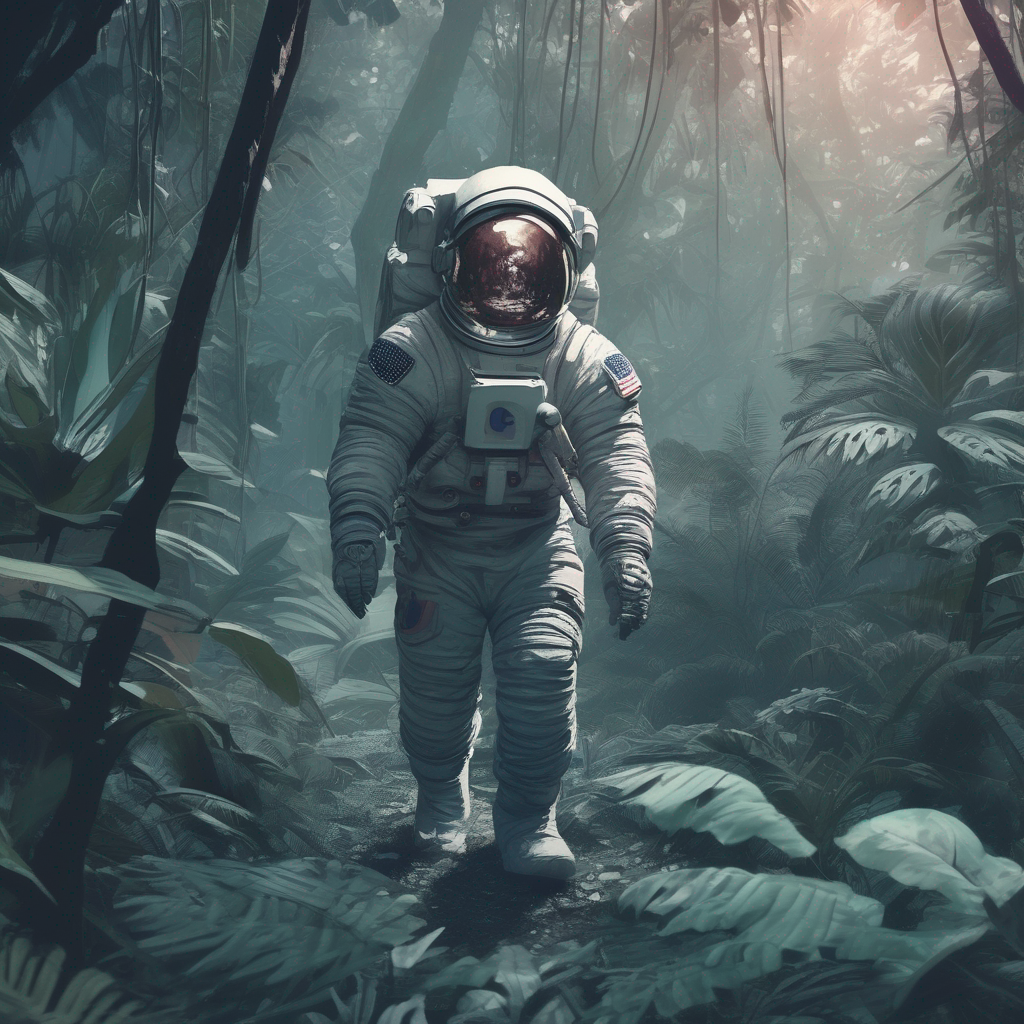

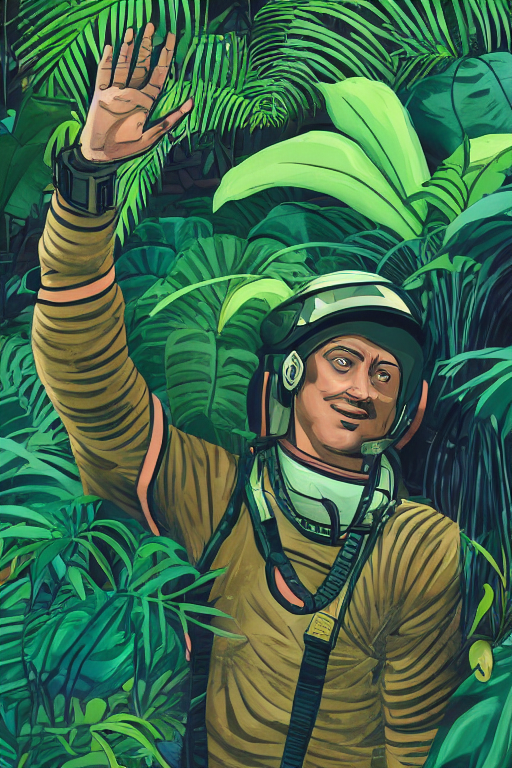
配置管道参数
管道中可以配置许多参数,这些参数会影响图像的生成方式。你可以更改图像的输出大小,指定负面提示以提高图像质量,等等。本节将深入探讨如何使用这些参数。
高度和宽度
height
和 width
参数控制生成图像的高度和宽度(以像素为单位)。默认情况下,Stable Diffusion v1.5 模型输出 512x512 图像,但你可以将其更改为 8 的倍数的任何大小。例如,要创建矩形图像:
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16, variant="fp16"
).to("cuda")
image = pipeline(
"Astronaut in a jungle, cold color palette, muted colors, detailed, 8k", height=768, width=512
).images[0]
image
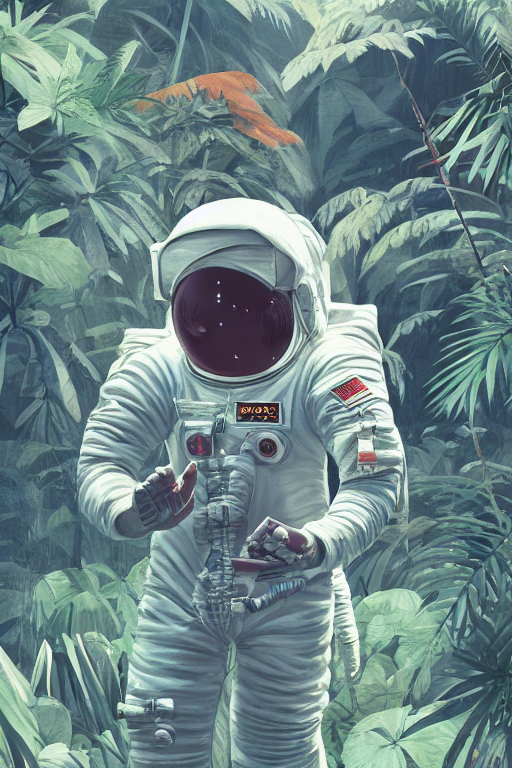
指导尺度
guidance_scale
参数影响提示对图像生成的程度。较低的值赋予模型"创造力",以生成与提示关系较松散的图像。较高的 guidance_scale
值会迫使模型更紧密地遵循提示,如果此值过高,你可能会在生成的图像中观察到一些伪影。
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16
).to("cuda")
image = pipeline(
"Astronaut in a jungle, cold color palette, muted colors, detailed, 8k", guidance_scale=3.5
).images[0]
image



负面提示
就像提示引导生成一样,负面提示引导模型远离你不希望模型生成的东西。这通常用于通过去除低分辨率或细节不佳等不良图像特征来提高整体图像质量。你也可以使用负面提示来删除或修改图像的内容和风格。
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16
).to("cuda")
image = pipeline(
prompt="Astronaut in a jungle, cold color palette, muted colors, detailed, 8k",
negative_prompt="ugly, deformed, disfigured, poor details, bad anatomy",
).images[0]
image


生成器
torch.Generator
对象通过设置手动种子来实现管道中的可重复性。你可以使用 Generator
生成图像批次,并根据 使用确定性生成提高图像质量 指南中所述,迭代地改进从种子生成的图像。
你可以在下面所示设置种子和 Generator
。使用 Generator
创建图像应该每次都返回相同的结果,而不是随机生成新图像。
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16
).to("cuda")
generator = torch.Generator(device="cuda").manual_seed(30)
image = pipeline(
"Astronaut in a jungle, cold color palette, muted colors, detailed, 8k",
generator=generator,
).images[0]
image
控制图像生成
除了配置管道的参数(例如提示词加权和 ControlNet 模型)之外,还有几种方法可以更好地控制图像的生成方式。
提示词加权
提示词加权是一种技术,用于增加或减少提示词中概念的重要性,以强调或最小化图像中的某些特征。我们建议使用 Compel 库来帮助你生成加权提示词嵌入。
创建完嵌入后,你可以将它们传递给管道中的 prompt_embeds
(如果你使用负面提示词,则传递给 negative_prompt_embeds
)参数。
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained(
"stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16
).to("cuda")
image = pipeline(
prompt_embeds=prompt_embeds, # generated from Compel
negative_prompt_embeds=negative_prompt_embeds, # generated from Compel
).images[0]
ControlNet
正如你在ControlNet部分中所见,这些模型通过整合额外的条件图像输入,提供了一种更灵活、更准确的图像生成方式。每个 ControlNet 模型都针对特定类型的条件图像进行预训练,以生成类似于它的新图像。例如,如果你使用一个针对深度图进行预训练的 ControlNet 模型,你可以将深度图作为条件输入提供给模型,它将生成一个保留其中空间信息的图像。这比在提示中指定深度信息更快、更容易。你甚至可以将多个条件输入与MultiControlNet结合起来!
你可以使用许多类型的条件输入,🤗 Diffusers 支持 Stable Diffusion 和 SDXL 模型的 ControlNet。查看更全面的ControlNet指南,了解如何使用这些模型。
优化
扩散模型很大,对图像进行去噪的迭代性质在计算上很昂贵且密集。但这并不意味着你需要访问强大的 - 甚至许多 - GPU 来使用它们。在消费级和免费层资源上运行扩散模型有很多优化技术。例如,你可以以半精度加载模型权重,以节省 GPU 内存并提高速度,或者将整个模型卸载到 GPU 上以节省更多内存。
PyTorch 2.0 还支持一种更节省内存的注意力机制,称为缩放点积注意力,如果你使用的是 PyTorch 2.0,它会自动启用。你可以将它与torch.compile
结合起来,使你的代码运行得更快:
from diffusers import AutoPipelineForText2Image
import torch
pipeline = AutoPipelineForText2Image.from_pretrained("stable-diffusion-v1-5/stable-diffusion-v1-5", torch_dtype=torch.float16, variant="fp16").to("cuda")
pipeline.unet = torch.compile(pipeline.unet, mode="reduce-overhead", fullgraph=True)